Background:
In my first year of undergrad, I worked at iD Tech Camp. I taught game design, Java programming and a few other technical and computer science classes to primarily middle school-aged students. Consequently, with a computer at their fingertips, many of these students would wander off on the internet or play computer games. To overcome this, I built a Java-based application that would connect to, at the time, this new service called Firebase and load various commands that I could insert on my version of the app. I could at the touch of a button close anyone who had a browser window open, blackout a computer screen, send a file to a computer and more.
Main concept:
In order to understand some of the concepts behind blockchain a bit better, I decided to make my own Python-based blockchain, with Flask as a REST API package, for peers on my network to communicate to each other.
I wanted to attempt the same concept as I did at iD Tech Camp, but using an immutable, distributed, database system (ie. Blockchain) to store my commands.
The framework behind the code is that if the seed URL does not currently host a blockchain (ie a valid REST request cannot return a blockchain JSON) then the genesis chain is created, and the peer gets a “TEACHER” role. The password for this role (using a sha256 library) is saved at that peer. Only a person with this username and password combination and this teacher role can add blocks to the chain.
Anyone who connects to this blockchain automatically gets assigned a “STUDENT” role, and anyone who connects to any peers on this chain also gets assigned a “STUDENT” role. Anyone can point a REST request at any peer on this chain to add a block (with the right user/password combination) with a list of commands and more.
When a block sits unconfirmed on a peer, the peer keeps “solving” it until it can be added to the blockchain. Here, since the point is not to solve a complex problem, like traditional blockchains, rather, the problem is simple as there is enough built-in security to make the chain immutable. Proof of Work: the sha256 library creates a hexadecimal string from the block object with a dynamic variable that changes until the hexadecimal string matches certain requirements. Since this problem is not too complex, it will not take more than one second. Once the peer has solved the block, it sends the block and the answer to everyone who is connected to it and so forth:
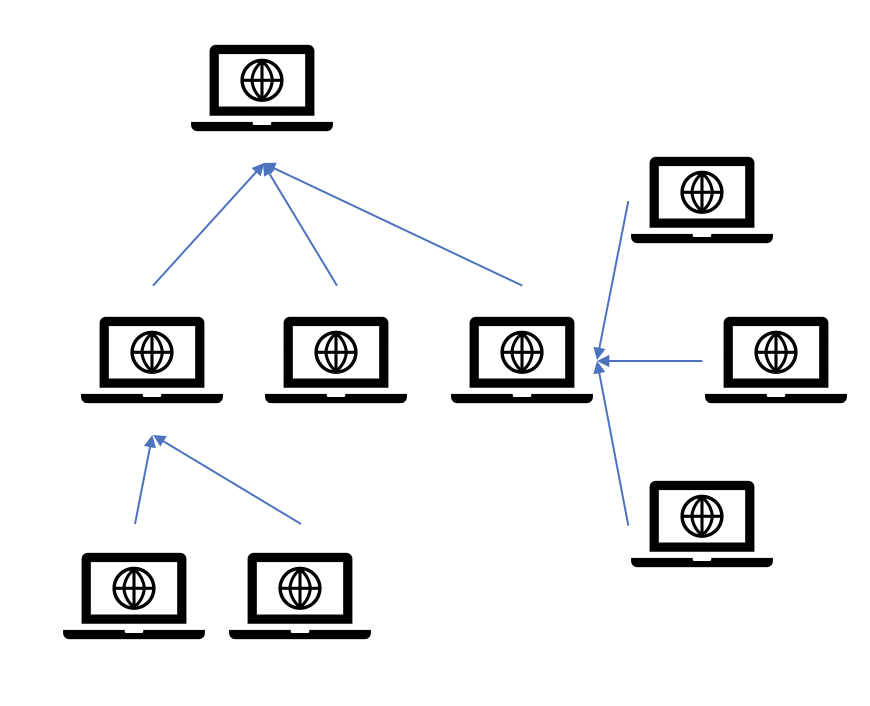
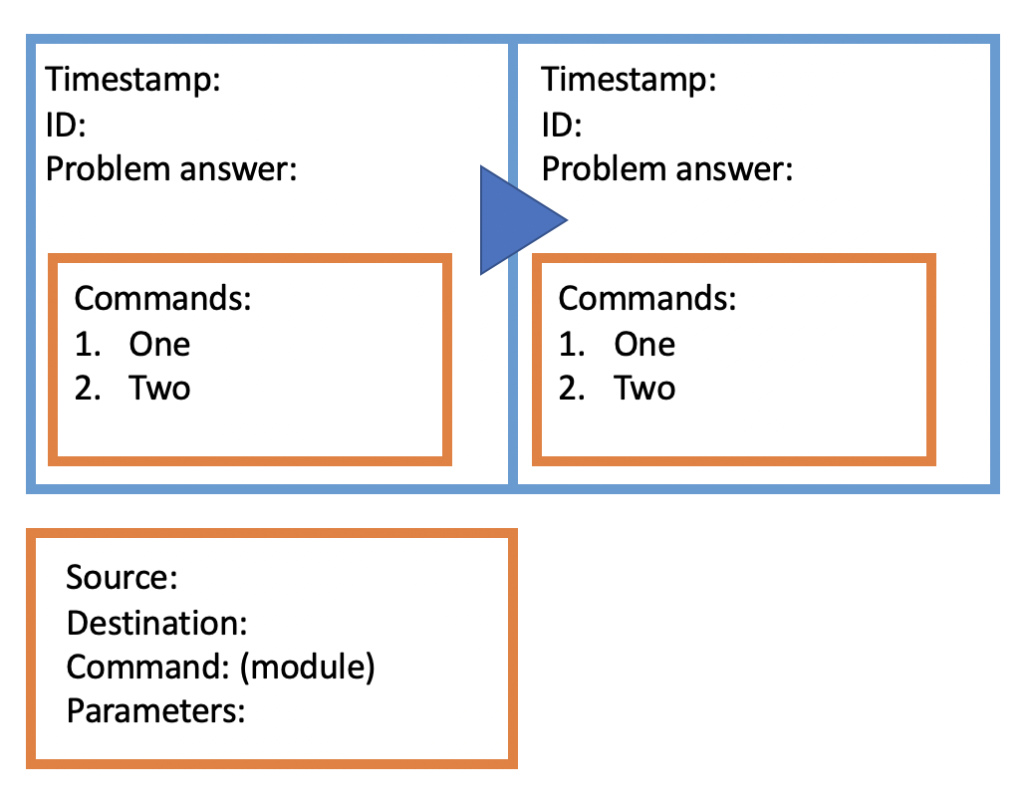
Built-in security and drawback:
The way authentication works on the chain is that every time a new peer (N) connects to an existing peer (E), that existing peer (E) now hosts the password for the new peer (N) on the chain. Whenever N needs to authenticate again, it can connect to any peer on the network, but these peers pass on the requests for authentication to the other peers until it reaches E. E then decides if the authentication is correct. This has built-in security because it means the password is delocalized – it is not stored in some central peer or select few peers. Furthermore, the password is never transmitted over the network, it is only known by E and the N.
The drawback is obvious, if E every goes offline, the peer N can no longer authenticate on the chain and must get a new identity. In future versions, the credentials could be stored in E as well as a trusted or random backup peer.
A modular approach to commands:
The code approaches commands in a modular way. A command can simply be written as a Python package and dropped in the ‘mods’ folder. This allows the opportunity to decide the level of complexity as well as ‘access’ each peer has on the network. It also allows peers to block the ability of the chain to run a command locally. It also makes it easier to add functionality and maintain the codebase. As long as a command with a module name matches a Python package in the ‘mods’ folder, the command can be executed.
Future:
In the future, I plan to make use of back up peers for hosting the password in case a peer goes offline. Additionally, I plan on using the ‘inspect’ features of Python to decide if a peer is trusted enough to connect. This can be used to ensure that the codebase that connects to the network is the same that the network is running.
The code is available on my GitHub: https://github.com/mhsiron/Crypto_CControl/